Reusable Components

v.18
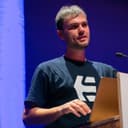
Fabio Biondi
Google Expert | Angular
1we will convert all Angular static components in dynamic reusable components
2we will use signal inputs and content projection
# Introduction
# Hero Component
We are currently using the Hero component in this way
However, the component template is static, so the content will always be the same if we try to use it elsewhere.
Our goal is to make it customizable by providing it custom title
, description
, image
, buttonLabel
and buttonUrl
An Angular component is just a class, and therefore we can pass properties to it, just like we would do in a regular class with setter methods:
The syntax to pass properties to an Angular component is slightly different but the concept is very similar:
In our scenario we want to pass all these properties:
The result:
Property Binding with brackets
In Angular, when brackets are not used, i.e. property="content"
, we're passing the "content" as a string.
When we use square brackets instead, i.e. [property]="content"
, we can pass expressions, in this case the content
value which may have been defined in the component class.
Brackets are then necessary if we want to pass arrays or object to a component input property.
Property binding (using []
) allows you to bind the values of component properties to the result of a template expression.
This means that the value of the property is dynamically determined and can change during the component's lifecycle.
When you need to pass complex data structures like objects or arrays to a component, property binding is the way to go.
The object { label: 'Start now', url: 'https://www.learnbydo.ing/' }
is a typical example of complex data.
Directly passing this object through property binding ensures that the app-hero
component receives the data in the correct format, otherwise it would have passed a string
# Create the Hero component
The Hero
component now looks like the following.
What we need to do is replace the static parts that we want to make dynamic with properties.
I'll highlight the parts we'll work on:
components/hero.component.ts
The first change I want to show you is to make the template dynamic so that it displays data from some properties that we will define in the component class.
components/hero.component.ts
I suggest you to read the previous chapters about Angular Fundamentals if you don't know what brackets are: {{...}}
or [...]
As you can see, now the values of the properties are displayed in the template.
Furthermore the button now displays the button.label
value and the URL is dynamic too since it binds button.url
# Make it reusable
The last change we did has created a dynamic template but you cannot still set them from the parent.
I mean, how can we set the properties in this way so we can use the app-hero
component passing different content?
# @Input decorator
Before Angular 16, the most common way to pass values from parent was by using the @Input
decorator that allowed us to define the properties that we want to expose:
components/hero.component.ts
With this update, it's now possible pass all properties from parent as attributes:
The HTML template of Hero component does not need to be changed since we have not changed the input properties names.
The only difference is that their values are passed by the parent rather than defined within it.
# Signal Inputs
But after version 16 we have a new way to allow values to be bound from parent components: signal inputs:
components/hero.component.ts
Previous code works exactly like the @Input
decorator, so we can pass properties in the exact same way.
But there is a big difference.
Now the Component's properties are signals
Accedi a TUTTI I CORSI PREMIUM ad un unico prezzo!