Store selectors

v.18

v.18
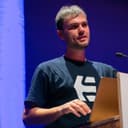
Fabio Biondi
Google Expert | Angular
# Introduction to Selectors
NGRX selectors allow you to extract and transform slices of the state in a performant and maintainable way.
Selectors are pure functions used to select, derive, and transform state from the NGRX store. They are typically used to:
- Extract specific pieces of state.
- Combine multiple pieces of state.
- Compute derived state based on one or more pieces of state.
# Why Use NGRX Selectors?
- Performance: selectors are memoized, meaning they remember the last result if the state hasn't changed, which helps in optimizing performance by avoiding unnecessary recalculations.
- Encapsulation: they encapsulate the logic of selecting and transforming state, making it reusable and easier to test.
- Composability: selectors can be composed together to create more complex selectors from simple ones.
# Write Selectors
Let's say we have this store:
The only way to read the store is by using selectors through the select
or selectSignal
functions provided by the Store
service. So:
- First we need to inject the
Store
And now we can read the values using select
:
[customName].selectors.ts
# Select State as Observable
Until NGRX 16, the state could only be retrieved as an Observable
using the .select
function as we have described in the previous recipe:
features/counter/counter.component.ts
async pipe or subscribe?
The async
pipe in Angular is a convenient and efficient way to handle and subscribe to an Observable directly within the template. It subscribes to an observable and returns the latest emitted value.
It means that the template always displays the latest state value, even when it changes.
When the component is destroyed, the async
pipe automatically unsubscribes to prevent potential memory leaks.
Of course you can also use the manual subscription to read the state in any class: counter$.subscribe(data => ...)
# Select State as a Signal
But after NGRX 16 we can also select a slice of the store as a Signal using selectSignal
and this is the way we'll use across the whole book, since signals offer an efficient and powerful way to handle local and global states and this is the recommended way in latest Angular versions:
features/counter/counter.component.ts
Currently the state is typed as any
so now you can make mistakes and write a wrong selector without compiler errors.
We will improve this in the next lessons, during which we will learn how to strongly type the state.
# Quiz Time
Which is the NGRX utility to select a portion of the store as a signal?
# Interactive Demo & Source Code
Accedi a TUTTI I CORSI PREMIUM ad un unico prezzo!