Notifications

v.7

v.18
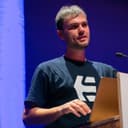
Fabio Biondi
Google Developer Expert
Microsoft MVP
# What are Notifications in RxJS?
In RxJS, Notifications are messages that represent the different types of events emitted by an Observable: next
, error
and completed
(and it is not a coincidence that they are the same properties defined by the Observer)
next
: represents a value emitted by the Observable.
The Marble diagram below shows an Observable that emits constant values over time and never completes, just like the interval
operator does.
How can we know that it never completes? There is no the |
symbol, that means complete
.
An Observable can emit multiple next
notifications over its lifecycle.
complete
: indicates that the Observable has finished emitting all its values.
The following Marble diagram shows an observable that emits three values over time (0
, 1
and 2
) and then complete
.
Looking at the diagram we can immediately understand that this Observable only emits 3 values and then complete
s.
How? Because there is a the vertical bar |
placed after the number 2
that indicate that it completes.
When a complete
notification is emitted, the Observable is considered done, and no further notifications (including next
) will be sent.
error
: represents an error that has occurred during the Observable's execution.
The diagram shows an error after the emission of the value 2
, by using the X
symbol
When an error
notification is emitted, the Observable's execution is interrupted, and no further notifications are sent.
It means that, just like for the complete
, after this notification there may be no more notifications of any kind.
Probably now this information will seem useless but it is a fundamental concept to understand when we combine more Observable, creating sequences.
# Example with interval
operator
Let’s start where we finished in the previous chapter:
In this scenario:
next
: emitted every seconds sending a value from 0
to N
error
: the interval
operator never generate an error, so this notification will never be emitted
complete
: the interval
never completes, so never emitted
However, it is not uncommon for a creation operator to never generate errors.
Imagine you have an observable that monitors the click
event of an button or the keydown
event of an input
text. These will never generate errors (of course if there is no other code that could affect their operations)
Instead an operator that makes an HTTP call to the server, as ajax
, could emit also an error
notification, or emits the complete
at the end.
There are ways to complete the interval
after a certain number of emissions (i.e. using the take(N)
pipeable operator which we will discuss later in the book) and it might also generates errors when combined with other RxJS operators.
# Example with ajax
operator
This following snippets use the ajax
operator to perform an HTTP GET request and fetches a JSON array of user data.
The diagram below shows two scenarios that could happen:
1The HTTP request completes successfully: a value is emitted (next
) and then completed (complete
)
2The HTTP request fails: an error notification is emitted (error
)