# Introduction
Zod is a TypeScript-first schema declaration and validation library that simplifies validations tasks with an elegant, developer-friendly API.
Born from the need for strong static typing, Zod allows developers to define the shape and constraints of their data using TypeScript's powerful type system.
Unlike other validation libraries that often require runtime type reflection or additional decorators, Zod leverages TypeScript’s compile-time features to ensure that data conforms to specified schemas without sacrificing performance.
In the world of software development, ensuring data integrity and validation is crucial, especially when dealing with complex applications.
Let me show you a simple example.
In the next snippet I use Zod to create a Schema and validate a string that should have a minimum of 3 characters and a maximum of 10.
You can use this approach to validate Forms, your HTTP response using fetch or Angular HttpClient, validate React Server actions and much more...

example.ts
<Practice>
Some snippets can be opened in a playground. Play with them!
Click or in the editor practice
This approach not only helps in catching errors early in the development cycle but also enhances code readability and maintainability.
Whether you're validating API responses, parsing client-side inputs, or structuring backend data processes, Zod provides a robust, efficient toolkit that seamlessly integrates into any TypeScript project.
By the end of this article, you’ll appreciate how Zod’s concise syntax and comprehensive features make it an indispensable tool for modern TypeScript applications, helping developers enforce data validation rules and build safer, more predictable software.
# Next.js & Zod
Next.js, a leading React framework, offers out-of-the-box features for server-side rendering, static site generation, API routes and more...
With the introduction of Next.js v. 13/14 , developers can leverage Zod to validate form data by using server actions or create custom route handlers (I mean endpoints and REST API) , ensuring that the data conforms to the expected schema before it's processed or sent to the backend.
This is particularly useful in scenarios like user registrations, settings changes, or any form-based interaction where data integrity is paramount.
Using Zod in Next.js can streamline client-side validations by defining a schema that automatically checks the type and format of incoming data.
This method reduces the amount of boilerplate code and increases the reliability of the application by catching errors early in the submission process.
Here’s a quick example of how you might use Zod to validate a simple user login REST API created in a Next.js application:


/api/login.api.ts
# Qwik & Zod
Qwik , a "new" fullstack framework known for its resume-able applications optimized for edge deployment, also integrates well with Zod to provide form validations. In Qwik, forms are a critical part of creating interactive applications, especially when dealing with user-generated content.
Zod's integration allows Qwik developers to define schemas that validate data on server but can also validate form inputs on the client side before they ever hit the server, greatly improving the user experience by ensuring that only valid data is processed.
The use of Zod in Qwik helps in maintaining a clean and manageable codebase, as validation logic is centralized and decoupled from UI logic. This makes the application easier to maintain and test
# Zod in Angular
Angular , a robust framework for building dynamic web applications, includes a well-equipped HTTP client for making requests to servers.
By leveraging Zod, developers can validate these server responses directly within Angular services or components, ensuring that the data structures received match the expected schemas.
This approach is a proactive defense mechanism that prevents potential bugs and errors that could arise from mismatched or malformed data.
- Type Safety: Zod enhances Angular's built-in type safety by validating the runtime data against predefined schemas, thereby ensuring that the data conforms to the specified types.
- Error Handling: By catching data errors as soon as a response is received, developers can handle them gracefully, providing better user feedback and avoiding runtime errors that might be harder to debug.
- Development Efficiency: Defining schemas for server responses makes the code more predictable and easier to understand. It reduces the need for manual checks scattered throughout the application.
# Installation

More info about installation and the whole documentation is available on Zod Website .
# Fetch Data in Vanilla JS
Now let me show you and example where we validate an object provided by the JSON Placeholder Users API website.
I'm using
fetch
but you can also axios
or any other strategies / libraries to fetch data.
example.ts
The response is just an object with an
User
, some primitives and nested object.
response
# Object Validation
How can we use this Schema to validate this object?
I won't explain describe every instruction because it's self-explanatory. For more information, consult the ZOD documentation .
Anyway, as you can see, you can validate each property, object or arrays by defining some validation rules:

example.ts
<Practice>
Click or in the editor practice
If we remove the comment in line 20 (where we check the
website
property), we can see how the parse
function of Zod generates an error:
The
safeParse
function would simply generates an object like this: {success: false }
that you can use to know if validation are passed or not# Quiz Time
Validate Fields
What is the right syntax to validate an email address?
z.mail()
z.email()
z.string().mail()
z.string().email()
# Array Validation
Of course we can also validate arrays.
First we load an array of
users
from JSON Placeholder Users API 
example.ts
We can then simply create a new Schema to validate the array:
- the array should contains
User
objects only - the array must not be empty
- it should have exactly 10 users
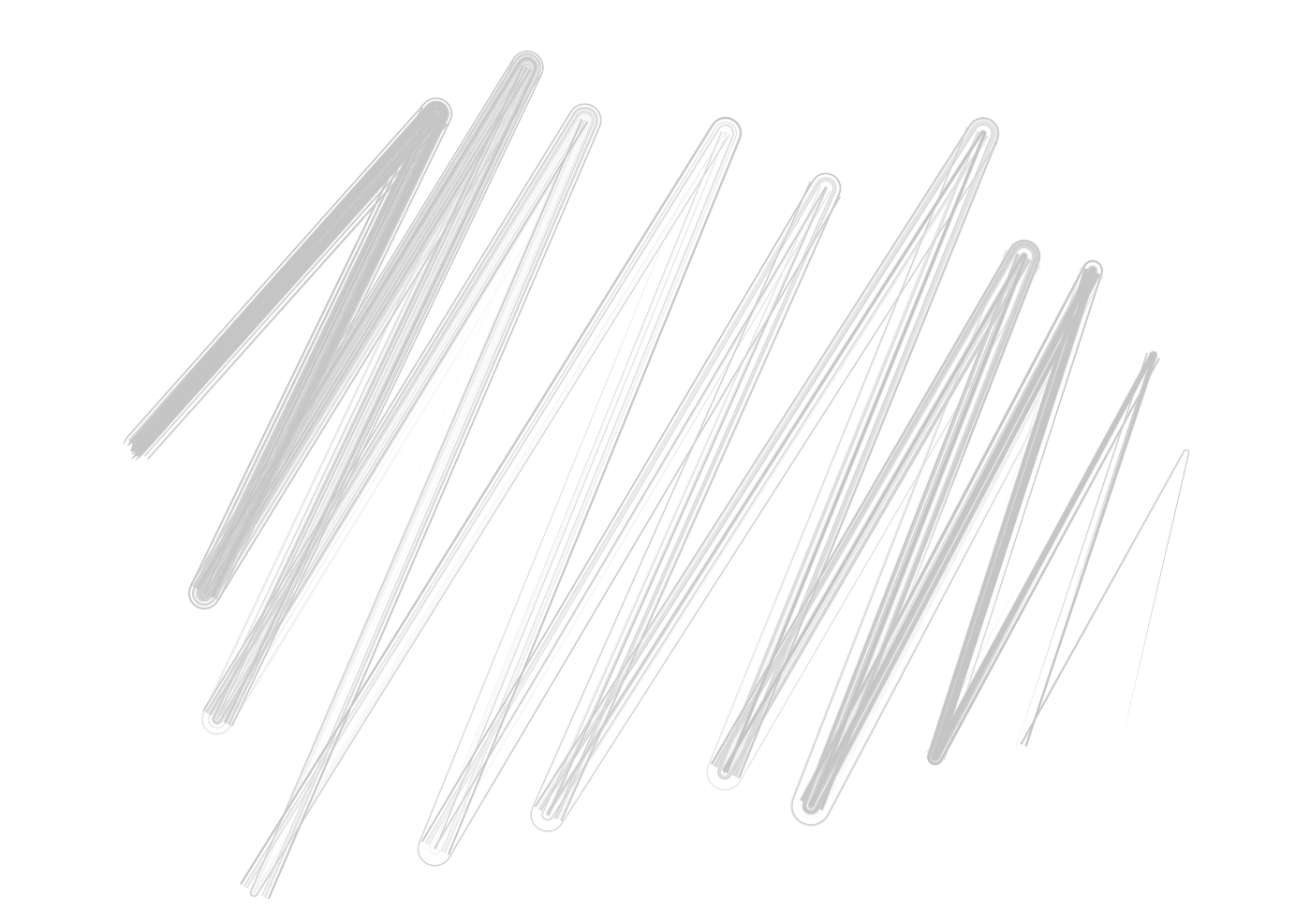